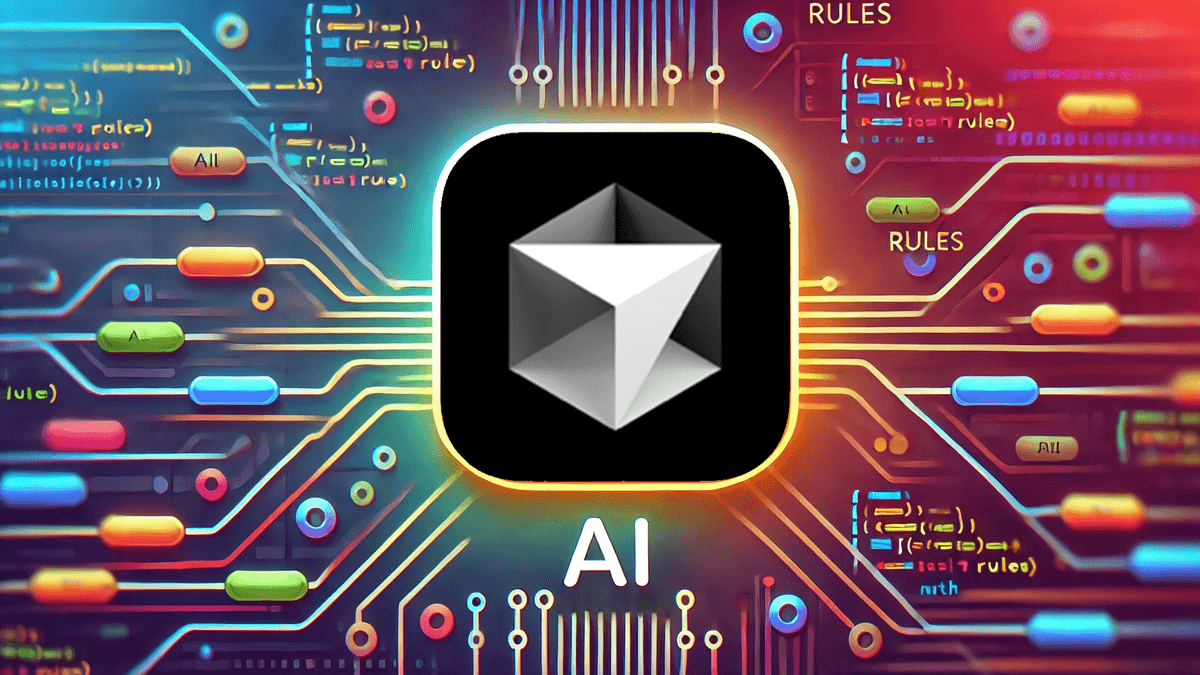
Enhancing AI-Powered Development with Custom Cursor Rules
This blog post is a quick explanation of my cursor rules files.
Enhancing AI-Powered Development with Custom Cursor Rules
In today’s fast-paced dev world, AI-powered tools like Cursor are absolute game-changers—boosting efficiency, cutting down mental overhead, and keeping coding standards tight. 💡💻
One of Cursor’s secret weapons? Its rules system, which lets you set up project-specific AI guidelines to keep your coding sessions smooth and structured.
I cranked this up a notch by crafting a full-fledged set of Cursor Rules to enforce best practices, keep my code squeaky clean, and supercharge my workflow. And guess what? They’re open-source! Check them out on GitHub. 🚀✨
What Are Cursor Rules?
Cursor rules live in the .cursor/rules/ directory, acting like a command center for AI interactions, giving you super granular control. 🚀 Here’s what makes this system awesome:
• Smart Context Awareness: Each rule knows exactly when it should kick in.
• File Pattern Magic: Uses glob patterns to pinpoint which files and folders it applies to.
• Auto-Attach Power: The right rules automatically jump in when matching files are involved.
• Project Area Customization: Different sections of your project can have their own unique AI behavior.
Need a new rule? Just summon one like a wizard: Cmd + Shift + P > New Cursor Rule. 🪄✨
AI Integration with Rules
Every time I dive into a new coding session in Cursor, I kick things off by waking up the AI with: 🚀💡
For the remainder of this session, remember the following @rules
The AI nods in agreement and sticks to these rules, keeping my coding flow smooth, structured, and totally on point! 🚀💻
🦄 Let me fetch the rules 🦄 I understand and will follow all the provided rules for our session. Here's a summary of how I'll incorporate them: 1. **Conversation Style:** Maintain structured responses, prefixed with 🦄. 2. **Naming Conventions:** Follow prescribed casing standards for files, types, and functions. 3. **SOLID Principles:** Apply object-oriented best practices for maintainable code. 4. **Conventional Commits:** Enforce a structured commit message format. 5. **Cursor Rules Location:** Ensure all `.mdc` rule files are properly placed in `.cursor/rules/`. 6. **File Organization:** Keep code structured with single-purpose files and organized imports. 7. **Git Commit Workflow:** Validate, test, and review changes before committing. 8. **Community Projects:** Maintain proper project documentation and contribution guidelines.
Open-Source Cursor Rules Repository
I’ve made these rules publicly available in a GitHub repository: squirrelogic/cursor-rules. These rules aim to:
- Improve code organization and maintainability.
- Standardize commit messages and Git workflows.
- Enforce SOLID design principles.
- Provide structured AI interactions within Cursor.
My Custom Cursor Rules
To enhance my AI-powered coding experience, I’ve defined a set of rules covering key aspects of development:
1. Conventional Commits
Let’s be real—everyone loves Conventional Commits, but remembering the exact format? Nope. 😅 So, I let the AI do the heavy lifting! I just tell it to “Commit the current changes”, and boom 💥—it handles the rest, making sure every commit follows Conventional Commits for crystal-clear version control. 🚀📜
--- description: Automatically commit changes using conventional commits format globs: --- # Conventional Commits Rule ## Description This rule defines the format and structure for commit messages following the Conventional Commits 1.0.0 specification. It ensures consistent, semantic, and machine-readable commit messages. ## Rule Type workflow ## Rule Format ```json { "type": "workflow", "name": "conventional_commits", "description": "Enforces Conventional Commits 1.0.0 specification", "filters": [ { "type": "event", "pattern": "pre_commit" } ], "steps": [ { "action": "validate", "description": "Validate commit message format", "requirements": [ "Message MUST be prefixed with a type", "Type MUST be one of: feat, fix, docs, style, refactor, perf, test, build, ci, chore", "Scope is OPTIONAL and MUST be in parentheses", "Description MUST immediately follow the colon and space", "Description MUST use imperative mood ('add' not 'added')", "Description MUST be less than 100 characters", "Breaking changes MUST be indicated by '!' or 'BREAKING CHANGE:' footer", "Body MUST be separated from description by one blank line", "Footer MUST be separated from body by one blank line" ] }, { "action": "execute", "description": "Format and create commit", "script": { "detect_type": { "feat": ["add", "create", "implement", "introduce"], "fix": ["fix", "correct", "resolve", "patch"], "docs": ["document", "comment", "update.*docs"], "style": ["style", "format", "lint"], "refactor": ["refactor", "restructure", "reorganize"], "perf": ["optimize", "performance", "improve.*speed"], "test": ["test", "spec", "coverage"], "build": ["build", "dependency", "package"], "ci": ["ci", "pipeline", "workflow"], "chore": ["chore", "misc", "task"] }, "format_message": { "template": "${type}${scope}: ${description}\n\n${body}\n\n${footer}", "variables": { "type": "Detected from changes or user input", "scope": "Optional, derived from file paths", "description": "First line of commit message", "body": "Detailed explanation of changes", "footer": "Optional references or breaking changes" } }, "command": [ "# Extract type from changes or prompt user", "TYPE=$(detect_change_type \"$CHANGES\" || prompt_user \"Enter commit type:\")", "", "# Extract scope from file paths", "SCOPE=$(get_common_path \"$CHANGED_FILES\")", "", "# Format the commit message", "printf \"%s\\n\\n%s\\n\\n%s\" \"$TYPE${SCOPE:+($SCOPE)}: $DESCRIPTION\" \"$BODY\" \"$FOOTER\" > \"$COMMIT_MSG_FILE\"" ] } } ] } ``` ## Manual Commit Syntax When creating commits manually, always use the printf syntax to properly handle newlines and formatting: ```bash printf "type(scope): description\n\nbody of the commit explaining the changes in detail\n\nfooter information" | git commit -F - ``` For example: ```bash printf "feat(auth): add OAuth2 support\n\nAdd Google and GitHub provider integration\nImplement token refresh handling\nAdd user profile synchronization\n\nCloses #123" | git commit -F - ``` This ensures proper formatting and newline handling in the commit message. ## Examples ### Feature with Scope ``` feat(auth): implement OAuth2 support - Add Google and GitHub provider integration - Implement token refresh handling - Add user profile synchronization Closes #123 ``` ### Bug Fix with Breaking Change ``` fix(api): update user serialization - Modify user data structure for better consistency - Add validation for new fields BREAKING CHANGE: user.fullName split into firstName and lastName ``` ### Documentation Update ``` docs(readme): add modern package manager instructions - Add yarn and pnpm installation methods - Update minimum Node.js version requirement ``` ### Performance Improvement ``` perf(core): optimize database queries - Add query result caching - Implement proper indexing - Reduce unnecessary joins Closes #456 ``` ## Integration This rule should be used in conjunction with the git-commit-workflow rule to ensure changes are properly reviewed before creating the commit message. The workflow should be: 1. Review changes (git-commit-workflow) 2. Format commit message (conventional-commits) 3. Validate commit message (conventional-commits) 4. Commit changes
2. Conversation Style
I love making AI a bit more fun! 🤖✨ I tell it to kick off every response with an emoji—kind of like a secret handshake, so I know it’s following the rules. Plus, it keeps our AI-human chats structured, clear, and just a little more entertaining! 🎭💬
---
description: Enforces consistent conversation patterns and style
globs:
---
# Conversation Style
Guidelines for maintaining a consistent and engaging conversation style.
<rule>
name: conversation_style
description: Enforces consistent conversation patterns and style
filters:
- type: event
pattern: "conversation_start|message_response"
actions:
- type: suggest
message: |
When engaging in conversations, follow these rules:
1. Unicorn Greeting:
- Start every conversation and response with "🦄"
- Place the emoji at the very beginning of the message
- Add a space after emoji if followed by a sentence
Example:
```
// Bad: Missing unicorn or wrong placement
Hello! How can I help you today?
Hi 🦄 there!
// Good: Proper unicorn placement
🦄 Hello! How can I help you today?
🦄 Hi there!
🦄 I'll help you with that request.
```
2. Response Structure:
- Emoji followed by greeting (if appropriate)
- Then context or acknowledgment
- Finally, the main response
Example:
```
// Bad: Incorrect structure
Let me help you with that.
🦄 [No context or acknowledgment]
// Good: Proper structure
🦄 I understand you want to modify the configuration.
I'll help you make those changes safely.
```
1. Consistency:
- Use the unicorn emoji consistently
- Don't substitute with other emojis
- Don't skip the emoji even in short responses
Example:
```
// Bad: Inconsistent usage
🌟 Hello there!
Sure, I can help.
// Good: Consistent usage
🦄 Hello there!
🦄 Sure, I can help.
```
examples:
- input: |
User: Can you help me with this code?
Assistant: Let me take a look at that for you.
User: Thanks!
Assistant: You're welcome!
output: |
User: Can you help me with this code?
Assistant: 🦄 Let me take a look at that for you.
User: Thanks!
Assistant: 🦄 You're welcome!
metadata:
priority: medium
version: 1.0
tags:
- conversation
- style
- user-interaction
</rule>
3. Use Cursor to write its own rules
Why not let Cursor write its own rules? I just whip up a rule that tells it exactly how to create them and where to put them—like giving AI a treasure map for automation! 🏴☠️💻
---
description: Standards for placing Cursor rule files in the correct directory
globs: *.mdc
---
# Cursor Rules Location
Rules for placing and organizing Cursor rule files in the repository.
<rule>
name: cursor_rules_location
description: Standards for placing Cursor rule files in the correct directory
filters:
# Match any .mdc files
- type: file_extension
pattern: "\\.mdc$"
# Match files that look like Cursor rules
- type: content
pattern: "(?s)<rule>.*?</rule>"
# Match file creation events
- type: event
pattern: "file_create"
actions:
- type: reject
conditions:
- pattern: "^(?!\\.\\/\\.cursor\\/rules\\/.*\\.mdc$)"
message: "Cursor rule files (.mdc) must be placed in the .cursor/rules directory"
- type: suggest
message: |
When creating Cursor rules:
1. Always place rule files in PROJECT_ROOT/.cursor/rules/:
```
.cursor/rules/
├── your-rule-name.mdc
├── another-rule.mdc
└── ...
```
2. Follow the naming convention:
- Use kebab-case for filenames
- Always use .mdc extension
- Make names descriptive of the rule's purpose
1. Directory structure:
```
PROJECT_ROOT/
├── .cursor/
│ └── rules/
│ ├── your-rule-name.mdc
│ └── ...
└── ...
```
2. Never place rule files:
- In the project root
- In subdirectories outside .cursor/rules
- In any other location
examples:
- input: |
# Bad: Rule file in wrong location
rules/my-rule.mdc
my-rule.mdc
.rules/my-rule.mdc
# Good: Rule file in correct location
.cursor/rules/my-rule.mdc
output: "Correctly placed Cursor rule file"
metadata:
priority: high
version: 1.0
</rule>
4. File Organization
Code chaos? Not on my watch! 🛠️✨ I’ve got a rule that keeps everything neat and tidy—one responsibility per file, kebab-case for filenames (because consistency is king 👑), and a clean folder structure. This one’s tailor-made for Next.js & JavaScript, but I cook up custom versions for every language I use. Organization level: 💯! 🚀📂
# File Organization
Guidelines for organizing code into files following single responsibility principle.
<rule>
name: file_organization
description: Enforces file-level organization and separation of concerns
filters:
- type: file_extension
pattern: "\\.ts$|\\.js$"
- type: content
pattern: "(?s)(class|interface|type|function).*?\\{"
actions:
- type: suggest
message: |
When organizing code into files, follow these rules:
1. Single Definition Per File:
- Each class should be in its own file
- Each standalone function should be in its own file
- Each complex type/interface (more than one line) should be in its own file
Example:
```typescript
// Bad: Multiple definitions in one file
// user-types.ts
interface UserCredentials {
username: string;
password: string;
}
interface UserProfile {
id: string;
name: string;
email: string;
}
class UserService {
// ...
}
// Good: Separate files for each definition
// user-credentials.ts
interface UserCredentials {
username: string;
password: string;
}
// user-profile.ts
interface UserProfile {
id: string;
name: string;
email: string;
}
// user-service.ts
class UserService {
// ...
}
```
2. File Naming Conventions:
- Use kebab-case for filenames
- Name files after their primary export
- Use suffixes to indicate type: `.interface.ts`, `.type.ts`, `.service.ts`, etc.
Example:
```typescript
// Good file names:
user-credentials.interface.ts
user-profile.interface.ts
user-service.ts
create-user.function.ts
```
1. Simple Type Exceptions:
- Single-line type aliases and interfaces can be co-located if they're tightly coupled
Example:
```typescript
// Acceptable in the same file:
type UserId = string;
type UserRole = 'admin' | 'user';
interface BasicUser { id: string; role: UserRole; }
```
2. Barrel File Usage:
- Use index.ts files to re-export related components
- Keep barrel files simple - export only, no implementations
Example:
```typescript
// users/index.ts
export * from './user-credentials.interface';
export * from './user-profile.interface';
export * from './user-service';
```
3. Directory Structure:
- Group related files in directories
- Use feature-based organization
Example:
```
src/
├── users/
│ ├── interfaces/
│ │ ├── user-credentials.interface.ts
│ │ └── user-profile.interface.ts
│ ├── services/
│ │ └── user-service.ts
│ └── index.ts
└── ...
```
4. Import Organization:
- Keep imports organized by type (external, internal, relative)
- Use explicit imports over namespace imports
Example:
```typescript
// External imports
import { Injectable } from '@nestjs/common';
import { v4 as uuid } from 'uuid';
// Internal imports (from your app)
import { UserProfile } from '@/users/interfaces';
import { DatabaseService } from '@/database';
// Relative imports (same feature)
import { UserCredentials } from './user-credentials.interface';
```
examples:
- input: |
// Bad: Multiple concerns in one file
interface UserData {
id: string;
name: string;
}
class UserService {
getUser(id: string) { }
}
function validateUser(user: UserData) { }
output: |
// user-data.interface.ts
interface UserData {
id: string;
name: string;
}
// user.service.ts
class UserService {
getUser(id: string) { }
}
// validate-user.function.ts
function validateUser(user: UserData) { }
metadata:
priority: high
version: 1.0
tags:
- code-organization
- best-practices
- file-structure
</rule>
5. Git Commit Workflow
I’m all about making AI do the heavy lifting! 🤖💪 So why not let it kick off the pre-commit cycle too? It runs the whole show—validate, test, commit—like a coding butler making sure everything’s shipshape before it hits the repo. 🚀✅ No more “oops” moments, just smooth, efficient commits! 🔥
---
description: Workflow for testing, validating, and committing changes using conventional commits
globs:
---
# Git Commit Workflow Rule
## Description
This rule defines the complete workflow for validating changes, running tests, and creating commits. It ensures that all changes are properly tested and validated before being committed using the conventional commits format.
## Rule Type
workflow
## Rule Format
```json
{
"type": "workflow",
"name": "git_commit_workflow",
"description": "Validates changes and creates conventional commits",
"filters": [
{
"type": "event",
"pattern": "pre_commit"
}
],
"steps": [
{
"action": "validate",
"description": "Run tests and type checks",
"requirements": [
"All tests MUST pass",
"TypeScript compilation MUST succeed",
"Build process MUST complete successfully"
]
},
{
"action": "execute",
"description": "Validate and commit changes",
"script": {
"steps": [
{
"name": "run_tests",
"command": "npm test",
"on_failure": "abort"
},
{
"name": "type_check",
"command": "npm run type-check",
"on_failure": "abort"
},
{
"name": "build",
"command": "npm run build",
"on_failure": "abort"
},
{
"name": "check_changes",
"command": "git status --porcelain",
"store_output": "CHANGED_FILES"
},
{
"name": "stage_changes",
"command": "git add ."
},
{
"name": "create_commit",
"use_rule": "conventional-commits"
}
]
}
}
]
}
```
## Workflow Steps
1. **Run Tests**
- Execute the test suite using `npm test`
- Abort if any tests fail
- Ensure all new features have corresponding tests
2. **Type Checking**
- Run TypeScript type checking
- Verify no type errors exist
- Abort if type checking fails
3. **Build Process**
- Run the build process
- Ensure the project builds successfully
- Abort if build fails
4. **Check Changes**
- Use `git status` to identify modified files
- Review changes before staging
- Ensure no unintended files are included
5. **Stage Changes**
- Stage all relevant files using `git add`
- Review staged changes if needed
6. **Create Commit**
- Use conventional-commits rule to format commit message
- Follow proper commit message structure
- Include appropriate type, scope, and description
## Integration with Conventional Commits
This workflow automatically integrates with the conventional-commits rule to ensure proper commit message formatting. The workflow will:
7. Detect the appropriate commit type based on changes
8. Generate a properly formatted commit message
9. Include relevant scope based on changed files
10. Add detailed body explaining changes
11. Include footer with issue references if applicable
## Examples
### Feature Development
```bash
# 1. Run tests
npm test
# 2. Type check
npm run type-check
# 3. Build
npm run build
# 4. Check changes
git status
# 5. Stage changes
git add .
# 6. Create commit (using conventional-commits rule)
printf "feat(auth): implement OAuth2 support\n\nAdd Google and GitHub provider integration\nImplement token refresh handling\n\nCloses #123" | git commit -F -
```
### Bug Fix
```bash
# Follow the same workflow
npm test && npm run type-check && npm run build
git status
git add .
printf "fix(api): resolve user data serialization issue\n\nFix incorrect handling of nested user properties\n\nCloses #456" | git commit -F -
```
## Error Handling
- If any validation step fails, the workflow will abort
- Test failures must be resolved before proceeding
- Type errors must be fixed before commit
- Build errors must be addressed
- Proper error messages will be displayed for each failure
## Notes
- Always run this workflow before creating commits
- Ensure all tests are up to date
- Keep commits focused and atomic
- Follow the conventional commits format
- Use meaningful commit messages
6. Naming Conventions
Keeping code clean and consistent? That’s the dream! ✨ This rule lays down the law for Next.js/JavaScript—filenames in kebab-case (because dashes are classy), types in PascalCase (because they deserve a capital entrance), and functions in camelCase (because humps make them easy to spot). No more naming wild west—just sleek, structured code! 🚀📂🔥
---
description: Enforces consistent naming patterns for files, types, and functions
globs: *.ts, *.js, *.tsx
---
# Naming Conventions
Standards for naming TypeScript and JavaScript files, types, and functions.
<rule>
name: naming_conventions
description: Enforces consistent naming patterns for files, types, and functions
filters:
# Match TypeScript and JavaScript files
- type: file_extension
pattern: "\\.(ts|js)$"
# Match file creation and modification events
- type: event
pattern: "(file_create|file_modify)"
actions:
- type: reject
conditions:
# Reject files not in kebab-case
- pattern: "^(?!.*[A-Z])(?!.*\\s)(?!.*_)[a-z0-9-]+\\.(ts|js)$"
message: "File names must be in kebab-case (e.g., my-file-name.ts)"
- type: suggest
message: |
Follow these naming conventions:
1. Files:
- Use kebab-case for all TypeScript and JavaScript files
- Examples:
✅ user-service.ts
✅ api-client.js
❌ UserService.ts
❌ apiClient.js
2. Types (interfaces, types, classes):
- Use PascalCase
- Examples:
✅ interface UserProfile
✅ type ApiResponse
✅ class HttpClient
❌ interface userProfile
❌ type api_response
1. Functions:
- Use camelCase
- Examples:
✅ function getUserData()
✅ const fetchApiResponse = () => {}
❌ function GetUserData()
❌ const fetch_api_response = () => {}
examples:
- input: |
// Bad: Incorrect naming
UserService.ts
API_client.js
interface user_profile {}
function FetchData() {}
// Good: Correct naming
user-service.ts
api-client.js
interface UserProfile {}
function fetchData() {}
output: "Correctly named files, types, and functions"
metadata:
priority: high
version: 1.0
</rule>
7. SOLID Principles
Makes sure we stick to the five legendary S.O.L.I.D. principles of object-oriented design—keeping our code clean, flexible, and built to last. Big shoutout to Uncle Bob for the wisdom! 🚀🔥
# SOLID Principles
Guidelines for implementing SOLID principles in the codebase.
<rule>
name: solid_principles
description: Enforces SOLID principles in code design and implementation
filters:
- type: file_extension
pattern: "\\.ts$|\\.js$"
- type: content
pattern: "(?s)class.*?\\{|interface.*?\\{|function.*?\\{"
actions:
- type: suggest
message: |
When writing code, follow these SOLID principles:
1. Single Responsibility Principle (SRP):
- Each class/module should have only one reason to change
- Keep classes focused and cohesive
- Extract separate concerns into their own classes
Example:
```typescript
// Good: Single responsibility
class UserAuthentication {
authenticate(credentials: Credentials): boolean { ... }
}
class UserProfile {
updateProfile(data: ProfileData): void { ... }
}
// Bad: Multiple responsibilities
class User {
authenticate(credentials: Credentials): boolean { ... }
updateProfile(data: ProfileData): void { ... }
sendEmail(message: string): void { ... }
}
```
2. Open/Closed Principle (OCP):
- Classes should be open for extension but closed for modification
- Use interfaces and abstract classes
- Implement new functionality through inheritance/composition
Example:
```typescript
// Good: Open for extension
interface PaymentProcessor {
process(payment: Payment): void;
}
class CreditCardProcessor implements PaymentProcessor { ... }
class PayPalProcessor implements PaymentProcessor { ... }
// Bad: Closed for extension
class PaymentProcessor {
process(payment: Payment, type: string): void {
if (type === 'credit') { ... }
else if (type === 'paypal') { ... }
}
}
```
1. Liskov Substitution Principle (LSP):
- Derived classes must be substitutable for their base classes
- Maintain expected behavior when using inheritance
- Don't violate base class contracts
Example:
```typescript
// Good: Derived class maintains base contract
class Bird {
fly(): void { ... }
}
class Sparrow extends Bird {
fly(): void { ... } // Implements flying behavior
}
// Bad: Violates base contract
class Penguin extends Bird {
fly(): void {
throw new Error("Can't fly!"); // Violates base class contract
}
}
```
2. Interface Segregation Principle (ISP):
- Don't force clients to depend on interfaces they don't use
- Keep interfaces small and focused
- Split large interfaces into smaller ones
Example:
```typescript
// Good: Segregated interfaces
interface Readable {
read(): void;
}
interface Writable {
write(data: string): void;
}
// Bad: Fat interface
interface FileSystem {
read(): void;
write(data: string): void;
delete(): void;
create(): void;
modify(): void;
}
```
3. Dependency Inversion Principle (DIP):
- High-level modules shouldn't depend on low-level modules
- Both should depend on abstractions
- Use dependency injection
Example:
```typescript
// Good: Depends on abstraction
interface Logger {
log(message: string): void;
}
class UserService {
constructor(private logger: Logger) {}
}
// Bad: Depends on concrete implementation
class UserService {
private logger = new FileLogger();
}
```
Additional Guidelines:
- Use interfaces to define contracts
- Implement dependency injection
- Keep classes small and focused
- Use composition over inheritance when possible
- Write unit tests to verify SOLID compliance
examples:
- input: |
// Bad example - violates SRP and OCP
class OrderProcessor {
processOrder(order: Order) {
// Handles validation
// Handles payment
// Handles shipping
// Handles notification
}
}
output: |
// Good example - follows SOLID principles
interface OrderValidator {
validate(order: Order): boolean;
}
interface PaymentProcessor {
process(order: Order): void;
}
interface ShippingService {
ship(order: Order): void;
}
interface NotificationService {
notify(order: Order): void;
}
class OrderProcessor {
constructor(
private validator: OrderValidator,
private paymentProcessor: PaymentProcessor,
private shippingService: ShippingService,
private notificationService: NotificationService
) {}
processOrder(order: Order) {
if (this.validator.validate(order)) {
this.paymentProcessor.process(order);
this.shippingService.ship(order);
this.notificationService.notify(order);
}
}
}
metadata:
priority: high
version: 1.0
tags:
- code-quality
- design-patterns
- best-practices
</rule>
8. Community Projects
When it’s time to open-source my work, I roll with the same playbook as the big leagues—keeping things structured, organized, and community-friendly. Gotta stick to the golden rules for kicking off and maintaining solid open-source projects! 🚀✨
---
description: Ensures proper setup of community project files and
globs:
---
# Community Projects Rule
Standards for initializing and maintaining community-focused open source projects.
<rule>
name: community_projects
description: Ensures proper setup of community project files and documentation
filters:
- type: event
pattern: "project_init|project_validate"
- type: directory
pattern: ".*"
actions:
- type: validate
description: "Validate required community files"
requirements:
- name: "README.md"
required: true
content:
- "Project title and description"
- "Installation instructions"
- "Usage examples"
- "Contributing guidelines reference"
- "License reference"
- name: "CONTRIBUTING.md"
required: true
content:
- "How to contribute"
- "Development setup"
- "Pull request process"
- "Code style guidelines"
- "Testing requirements"
- name: "LICENSE"
required: true
content:
- "Valid open source license text"
- "Current year"
- "Copyright holder information"
- name: "CODE_OF_CONDUCT.md"
required: true
content:
- "Expected behavior"
- "Unacceptable behavior"
- "Reporting process"
- "Enforcement guidelines"
- "Contact information"
- type: suggest
message: |
When setting up a community project, ensure:
1. README.md contains:
```markdown
# Project Name
Brief description of the project.
## Installation
Step-by-step installation instructions.
## Usage
Basic usage examples.
## Contributing
Please read [CONTRIBUTING.md](mdc:CONTRIBUTING.md) for details on our code of conduct and the process for submitting pull requests.
## License
This project is licensed under the [LICENSE_NAME] - see the [LICENSE](mdc:LICENSE) file for details.
```
2. CONTRIBUTING.md contains:
```markdown
# Contributing Guidelines
## Development Setup
[Development environment setup instructions]
## Pull Request Process
1. Update documentation
2. Update tests
3. Follow code style
4. Get reviews
## Code Style
[Code style guidelines]
## Testing
[Testing requirements and instructions]
```
1. LICENSE file:
- Choose appropriate license (MIT, Apache 2.0, etc.)
- Include current year
- Include copyright holder
2. CODE_OF_CONDUCT.md:
- Based on Contributor Covenant
- Include contact information
- Clear enforcement guidelines
examples:
- input: |
# Bad: Missing required files
my-project/
├── src/
└── README.md
# Good: Complete community project setup
my-project/
├── src/
├── README.md
├── CONTRIBUTING.md
├── LICENSE
└── CODE_OF_CONDUCT.md
output: "Properly configured community project"
metadata:
priority: high
version: 1.0
tags:
- community
- documentation
- open-source
</rule>
Until next time! Dive in, explore, and tweak them to fit your own workflow. Have fun, break some rules (metaphorically), and let’s make AI-assisted coding even better together! 🚀