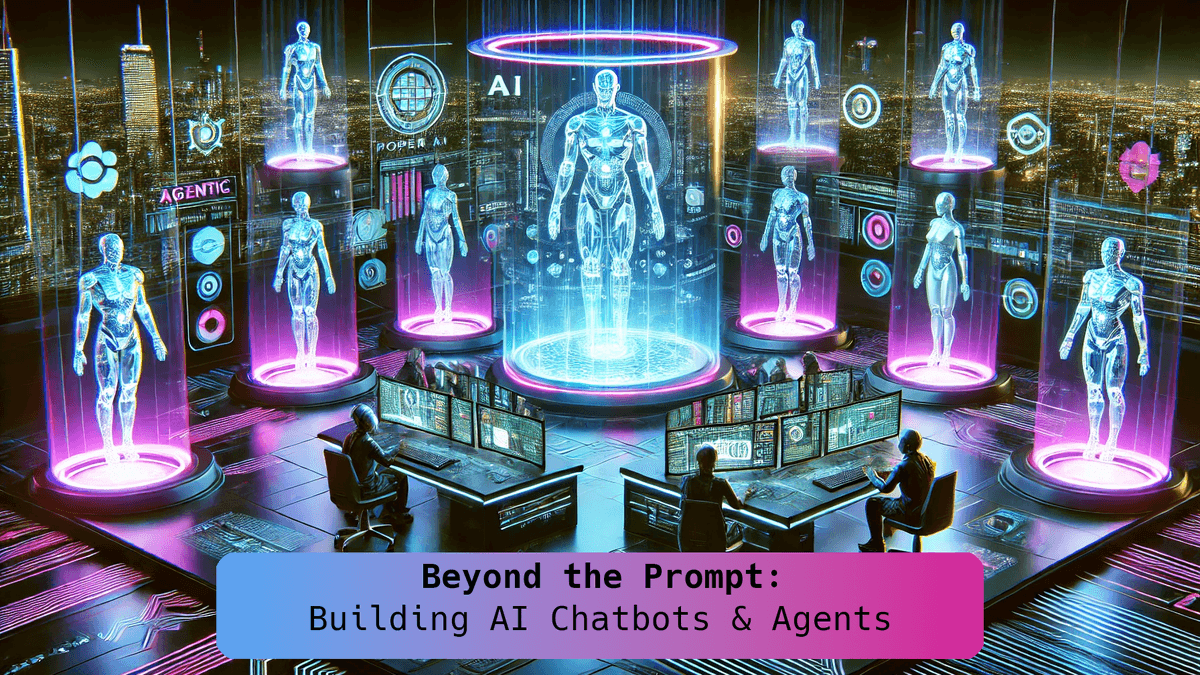
Introduction to LLMs π§ and Chatbots π¬ in Python π
This blog post serves as an introduction to Large Language Models (LLMs) and chatbots in Python, providing foundational knowledge for building AI-powered conversational agents.
What are LLMs, and How Do They Work? π€
Large Language Models (LLMs) are advanced AI π€ models trained on massive amounts of π text data to generate human-like responses. These models, such as OpenAI's π’ GPT-4, Anthropic's π€ Claude, and DeepSeek, utilize deep learning π§ techniques, specifically transformer β‘ architectures, to process and generate text based on input prompts.
Key Components of LLMs ποΈ:
- Tokenization π : LLMs break down text into smaller units called tokens, which can be words or subwords.
- Training Data π: These models are trained on diverse datasets, including books, articles, and online conversations.
- Neural Network Architecture πΈοΈ: They rely on transformer-based architectures, using self-attention π§ mechanisms to understand context and generate relevant responses.
- Inference & Response Generation π‘: Given an input prompt, the model predicts the next most likely tokens, forming coherent and meaningful replies.
Applications of LLMs in Chatbots π€:
- Conversational Assistants π£οΈ: AI-powered chatbots that answer questions, provide recommendations, or assist with tasks.
- Customer Support π§: Automated responses for handling common inquiries in businesses.
- Personalized AI Agents π€: Interactive tools that can remember context and assist users over time.
Hosted vs. Local Models: Why Start with Cloud APIs? βοΈ
When developing chatbots or AI agents, you can either use hosted (cloud-based) models via APIs or run models locally. Hereβs a comparison:
Feature π·οΈ | Cloud-Based APIs βοΈ (e.g., OpenAI, Anthropic, DeepSeek) | Local Models π₯οΈ (e.g., Hugging Face, Ollama) |
---|---|---|
Ease of Use ποΈ | Simple API calls, no setup required | Requires installation and setup π οΈ |
Performance β‘ | High performance, optimized infrastructure | Dependent on local hardware π₯οΈ |
Cost π° | Pay per use (can be expensive at scale) | Free after setup, but needs resources π |
Customization π¨ | Limited fine-tuning options | Full control over fine-tuning π οΈ |
Latency β³ | Low latency with cloud infrastructure | May experience delays based on hardware π |
Why Start with Cloud APIs? βοΈ
- π Quick setup and easier development.
- π― Access to high-performance, state-of-the-art models.
- ποΈ No need for expensive hardware or model training expertise.
- π Flexibility to switch providers without worrying about infrastructure.
Setting Up Your Python Environment π
Before we start building chatbots, we need a proper Python development environment.
Step 1: Install Python π₯οΈ
Ensure you have Python installed (version 3.8 or later). You can check your version with:
python --version
If Python is not installed, download it from python.org.
Step 2: Create a Virtual Environment π οΈ
Using a virtual environment helps manage dependencies cleanly. To create one:
# Create a virtual environment named 'chatbot_env' python -m venv chatbot_env # Activate the virtual environment # On Windows πͺ chatbot_env\Scripts\activate # On macOS/Linux π§ source chatbot_env/bin/activate
Step 3: Upgrade Pip β«
Ensure your package manager is up to date:
pip install --upgrade pip
Installing the OpenAI Python Library π€
To interact with OpenAIβs models, install their official Python package:
pip install openai
To verify installation, try importing OpenAI in Python:
import openai print("OpenAI library installed successfully! π")
Running Your First LLM-Powered Chatbot π
Now that our environment is set up, let's create a Python script to test an LLM.
Create a new file called chatbot.py
and add the following code:
import openai
openai.api_key = "your-api-key-here"
def chat_with_llm(prompt):
response = openai.ChatCompletion.create(
model="gpt-4",
messages=[{"role": "user", "content": prompt}]
)
return response["choices"][0]["message"]["content"]
if __name__ == "__main__":
user_input = input("Ask the AI: ")
reply = chat_with_llm(user_input)
print("AI:", reply)
Save the file and run it using:
python chatbot.py
This script allows you to interact with the LLM from your terminal. In the next article, we will enhance this chatbot further! π