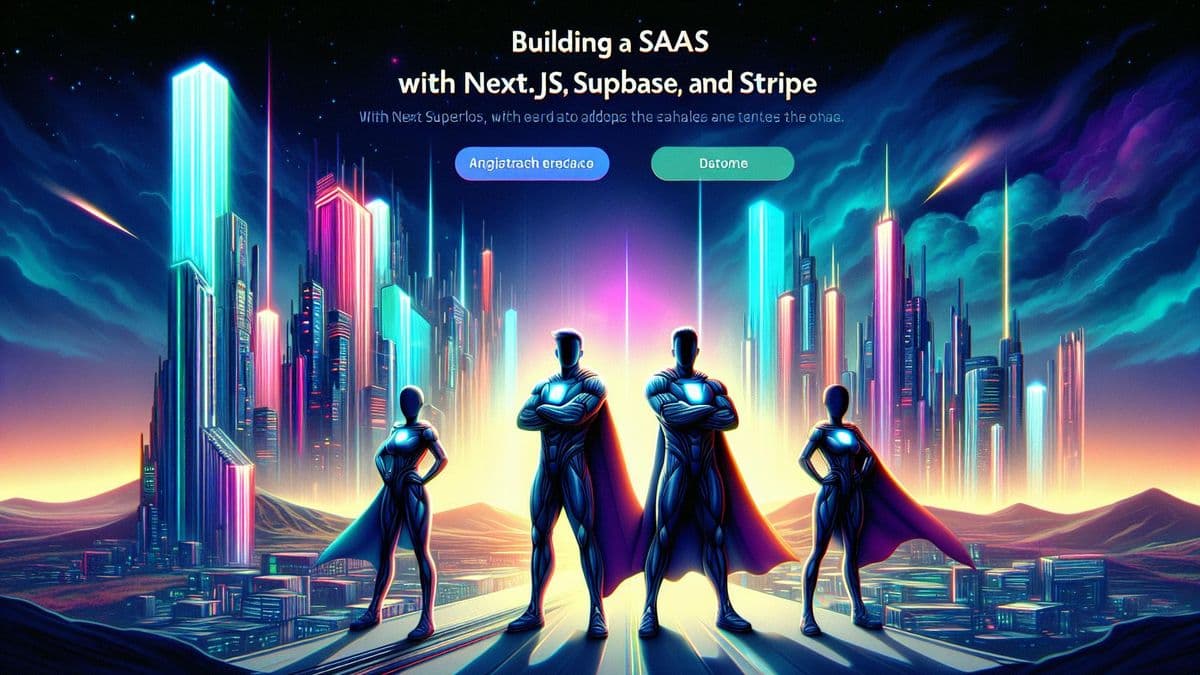
Building a SaaS with Next.js, Supabase, and Stripe
A guide to building a SaaS application using Next.js, Supabase, and Stripe, showcasing how this powerful stack enables seamless authentication, real-time database management, and subscription-based payments
Building a Software as a Service (SaaS) application today is like being Bruce Wayne in a sea of Alfreds—there's a powerful tech stack jam-packed with exciting gadgets to help you get the job done smoothly. In this post, we'll explore building a SaaS using Next.js, Supabase, and Stripe: a superhero trio that's as mighty as it sounds for creating scalable and efficient web applications.
Whether you're embarking on this journey for the exhilarating code adventures or just looking to expand your tech repertoire, let's navigate the practical and theoretical angles of pulling off this mission.
Why These Three Musketeers?
To craft a full-stack SaaS, you need tools that are agile and pack a punch. That's why we have Next.js, Supabase, and Stripe in our arsenal:
Next.js
- Server-Side Rendering (SSR) and Static Site Generation (SSG): Build fast, performant web apps with ease.
- React-based Framework: If you're familiar with React, Next.js feels like slipping into a comfy suit.
Supabase
- Real-time Database: Think Firebase, but open-source and with a hint of swagger. Great for real-time communication.
- Authentication & Storage Solutions: It's like having a trusty butler taking care of your backend needs seamlessly.
Stripe
- Seamless Payment Integration: It’s the gold standard for secure and efficient payment processing.
- Handles Complex Pricing Models: Subscriptions, one-time purchases, you name it—Stripe's got your back.
Setting Sail on Your Development Journey
Building a SaaS with this stack involves several key steps, each essential to ensuring our app is battle-ready. Buckle up as we dive deep into it.
Step 1: Setting Up Your Next.js Environment
Start by setting up the Next.js environment. It's like preparing your Batcave before taking on the night. Use create-next-app
to scaffold out your project:
npx create-next-app my-awesome-saas cd my-awesome-saas
This command whips up a fresh Next.js project, ready to be molded into something spectacular. Customize the homepage, set up routes, and incorporate server-side props as per your needs.
Step 2: Tuning in with Supabase
Time to lay the groundwork and set up Supabase, which will be your backend-as-a-service.
Authentication
Supabase makes integrating authentication a breeze—think of it as having Alfred to manage the door while you're on a mission.
-
Setting Up Authentication:
Create your Supabase project and configure authentication from the dashboard. Use pre-built methods for logging in with email, passwords, or OAuth.
-
Integration with Next.js:
Add Supabase's client library:
npm install @supabase/ssr @supabase/supabase-js
In your Next.js app, set up a Supabase browser client:
import { createBrowserClient } from '@supabase/ssr'
export function createClient() {
return createBrowserClient(
process.env.NEXT_PUBLIC_SUPABASE_URL!,
process.env.NEXT_PUBLIC_SUPABASE_ANON_KEY!
)
}
Then setup a Supabase server client
import { createServerClient } from '@supabase/ssr'
import { cookies } from 'next/headers'
export async function createClient() {
const cookieStore = await cookies()
return createServerClient(
process.env.NEXT_PUBLIC_SUPABASE_URL!,
process.env.NEXT_PUBLIC_SUPABASE_ANON_KEY!,
{
cookies: {
getAll() {
return cookieStore.getAll()
},
setAll(cookiesToSet) {
try {
cookiesToSet.forEach(({ name, value, options }) =>
cookieStore.set(name, value, options)
)
} catch {
// The `setAll` method was called from a Server Component.
// This can be ignored if you have middleware refreshing
// user sessions.
}
},
},
}
)
}
Then create the Supabase middleware
import { createServerClient } from '@supabase/ssr'
import { NextResponse, type NextRequest } from 'next/server'
export async function updateSession(request: NextRequest) {
let supabaseResponse = NextResponse.next({
request,
})
const supabase = createServerClient(
process.env.NEXT_PUBLIC_SUPABASE_URL!,
process.env.NEXT_PUBLIC_SUPABASE_ANON_KEY!,
{
cookies: {
getAll() {
return request.cookies.getAll()
},
setAll(cookiesToSet) {
cookiesToSet.forEach(({ name, value, options }) => request.cookies.set(name, value))
supabaseResponse = NextResponse.next({
request,
})
cookiesToSet.forEach(({ name, value, options }) =>
supabaseResponse.cookies.set(name, value, options)
)
},
},
}
)
// Do not run code between createServerClient and
// supabase.auth.getUser(). A simple mistake could make it very hard to debug
// issues with users being randomly logged out.
// IMPORTANT: DO NOT REMOVE auth.getUser()
const {
data: { user },
} = await supabase.auth.getUser()
if (
!user &&
!request.nextUrl.pathname.startsWith('/login') &&
!request.nextUrl.pathname.startsWith('/auth')
) {
// no user, potentially respond by redirecting the user to the login page
const url = request.nextUrl.clone()
url.pathname = '/login'
return NextResponse.redirect(url)
}
// IMPORTANT: You *must* return the supabaseResponse object as it is.
// If you're creating a new response object with NextResponse.next() make sure to:
// 1. Pass the request in it, like so:
// const myNewResponse = NextResponse.next({ request })
// 2. Copy over the cookies, like so:
// myNewResponse.cookies.setAll(supabaseResponse.cookies.getAll())
// 3. Change the myNewResponse object to fit your needs, but avoid changing
// the cookies!
// 4. Finally:
// return myNewResponse
// If this is not done, you may be causing the browser and server to go out
// of sync and terminate the user's session prematurely!
return supabaseResponse
}
Now create a middleware.ts at the root of your project
import { type NextRequest } from 'next/server'
import { updateSession } from '@/utils/supabase/middleware'
export async function middleware(request: NextRequest) {
return await updateSession(request)
}
export const config = {
matcher: [
/*
* Match all request paths except for the ones starting with:
* - _next/static (static files)
* - _next/image (image optimization files)
* - favicon.ico (favicon file)
* Feel free to modify this pattern to include more paths.
*/
'/((?!_next/static|_next/image|favicon.ico|.*\\.(?:svg|png|jpg|jpeg|gif|webp)$).*)',
],
}
-
Secure User Management:
With Supabase hooked up, create a login server action
'use server'
import { revalidatePath } from 'next/cache'
import { redirect } from 'next/navigation'
import { createClient } from '@/utils/supabase/server'
export async function login(formData: FormData) {
const supabase = await createClient()
// type-casting here for convenience
// in practice, you should validate your inputs
const data = {
email: formData.get('email') as string,
password: formData.get('password') as string,
}
const { error } = await supabase.auth.signInWithPassword(data)
if (error) {
redirect('/error')
}
revalidatePath('/', 'layout')
redirect('/')
}
export async function signup(formData: FormData) {
const supabase = await createClient()
// type-casting here for convenience
// in practice, you should validate your inputs
const data = {
email: formData.get('email') as string,
password: formData.get('password') as string,
}
const { error } = await supabase.auth.signUp(data)
if (error) {
redirect('/error')
}
revalidatePath('/', 'layout')
redirect('/')
}
Step 3: Crafting the Database & Handling Storage
Leverage Supabase's real-time databases for your CRUD operations and data storage.
- Data Modeling: Configure your tables on the Supabase dashboard, applying row-level security (RLS) to ensure each user accesses only their data.
- Operations in Next.js: Execute CRUD operations using Supabase client:
// Example: Fetch all items const fetchItems = async () => { const { data, error } = await supabase .from('items') .select(); if (error) console.error(error); return data; };
Step 4: Subscription Management with Stripe
No SaaS adventure is complete without handling payments, and here enters Stripe.
Stripe Setup
-
Install Stripe: Integrate Stripe into Next.js:
npm install stripe
-
Create a Billing Page:
Set up a simple payment form using Stripe Elements. Here's a basic setup:
// components/Checkout.js import { useStripe, useElements, CardElement } from '@stripe/react-stripe-js'; const CheckoutForm = () => { const stripe = useStripe(); const elements = useElements(); const handleSubmit = async (event) => { event.preventDefault(); if (!stripe || !elements) return; const card = elements.getElement(CardElement); const { error, paymentMethod } = await stripe.createPaymentMethod({ type: 'card', card: card, }); if (error) { console.error(error); } else { console.log(paymentMethod); // Implement further payment processing logic } }; return ( <form onSubmit={handleSubmit}> <CardElement /> <button type="submit" disabled={!stripe}>Pay</button> </form> ); };
-
Server-side Configuration:
Use Next.js API routes to manage subscription logic. Ensure sensitive keys are kept server-side to maintain security integrity.
Step 5: Deploying Your SaaS Superstar
Once everything is polished, deploy your application. Platforms like Vercel and Netlify offer easy deployments for Next.js apps, with effortless scaling.
Ensuring Robust Security
Make security your top sidekick by enforcing rigorous access controls, using HTTPS, and routinely updating dependencies. With Supabase and Stripe, you're already starting strong on this front.
Adding Flair with Integrations
Consider enhancing your app's capabilities with integrations. From AI-enhanced features using services like Replicate to user analytics, the sky's the limit!
Wrapping Things Up
Navigating the realms of Next.js, Supabase, and Stripe brings the sophisticated prowess of modern full-stack development right at your fingertips. Not only do we gain a robust tech stack, but also mold it into a solution that's resilient, scalable, and ready for prime time.
With this hands-on guide, you're now equipped to embark on the triumphant journey of SaaS creation. Remember, the tools are mighty, but it's the coder with the plan who creates magic. Let the coding commence; your great SaaS awaits!
References and Further Reading
Dive deeper with these resources:
- Next.js Documentation
- Supabase Docs
- Stripe Developer Docs
- Community gems on GitHub and Medium
That’s it for now. Happy coding, and may your app be as bug-free as a January morning! 🍀