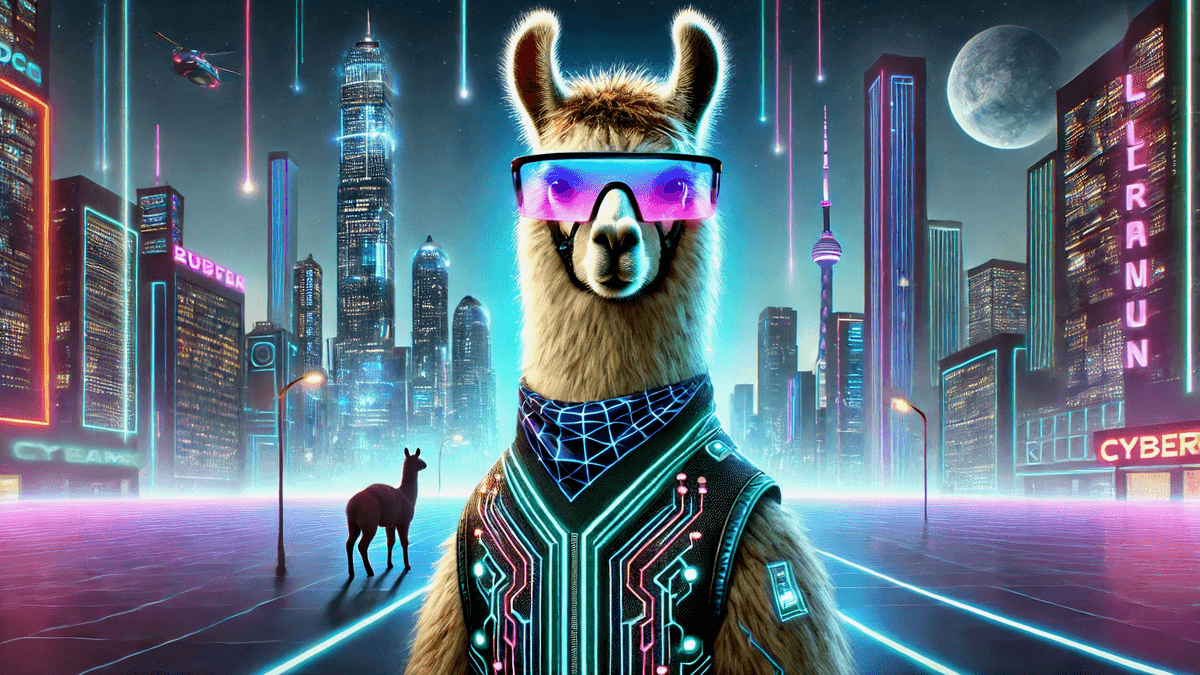
Running Open-Source LLMs Locally with Ollama and 4-bit Quantization
A practical guide to running powerful language models on your own hardware using Ollama and 4-bit quantization techniques.
Running large language models locally has become increasingly accessible thanks to tools like Ollama and advanced quantization techniques. In this guide, we'll explore how to run powerful LLMs on your own hardware efficiently.
Why Run LLMs Locally?
There are several compelling reasons to self-host language models:
- Cost efficiency for high-volume usage
- Data privacy and security
- Offline capability
- Full control over model behavior
- No API rate limits
Getting Started with Ollama
First, let's install Ollama:
# macOS or Linux curl https://ollama.ai/install.sh | sh # Verify installation ollama --version
Available Models
Ollama provides access to various optimized models:
-
Mistral
- Excellent performance-to-size ratio
- Great for general tasks
- ~7B parameters
-
Llama2
- Multiple sizes (7B, 13B, 70B)
- Strong reasoning capabilities
- Good coding assistant
-
CodeLlama
- Specialized for programming
- Better code completion
- Available in multiple sizes
Using 4-bit Quantization
4-bit quantization dramatically reduces model size while maintaining performance:
from transformers import AutoModelForCausalLM, AutoTokenizer
import torch
def load_quantized_model(model_name):
# Load the model with 4-bit quantization
model = AutoModelForCausalLM.from_pretrained(
model_name,
device_map="auto",
load_in_4bit=True,
bnb_4bit_quant_type="nf4",
bnb_4bit_compute_dtype=torch.float16
)
return model
# Example usage
model_name = "mistralai/Mistral-7B-v0.1"
model = load_quantized_model(model_name)
Running Models with Ollama
Basic usage with the Ollama API:
import requests
def query_model(prompt, model="mistral"):
response = requests.post('http://localhost:11434/api/generate',
json={
'model': model,
'prompt': prompt,
'stream': False
})
return response.json()['response']
# Example usage
result = query_model("Explain quantum computing in simple terms")
print(result)
Performance Optimization Tips
-
Memory Management
- Use appropriate model sizes for your hardware
- Monitor RAM usage during inference
- Consider batch processing for multiple requests
-
Speed Optimization
- Enable GPU acceleration when available
- Use efficient prompt templates
- Implement response streaming for better UX
-
Model Selection
- Choose models based on your specific use case
- Consider the trade-off between size and performance
- Test different quantization levels
Custom Model Configuration
Create a custom Modelfile for specific requirements:
FROM mistral:latest # Set parameters PARAMETER temperature 0.7 PARAMETER top_p 0.9 PARAMETER top_k 50 # Custom system prompt SYSTEM """ You are a helpful AI assistant focused on providing clear and concise responses. Please be direct and factual in your answers. """
Monitoring and Maintenance
Keep your local LLM deployment healthy:
-
Resource Monitoring
- Track CPU/GPU usage
- Monitor memory consumption
- Log inference times
-
Regular Updates
- Keep Ollama up to date
- Check for model updates
- Update quantization parameters
-
Error Handling
- Implement robust error handling
- Set up automatic restarts
- Monitor API endpoint health
Conclusion
Running LLMs locally with Ollama and 4-bit quantization provides a powerful, cost-effective solution for AI applications. The combination of efficient quantization and Ollama's user-friendly interface makes it accessible to developers of all skill levels.
Stay tuned for more advanced topics like:
- Fine-tuning local models
- Advanced prompt engineering
- Deployment strategies
- Performance benchmarking
Remember to always consider your specific use case when choosing between local deployment and cloud-based solutions.