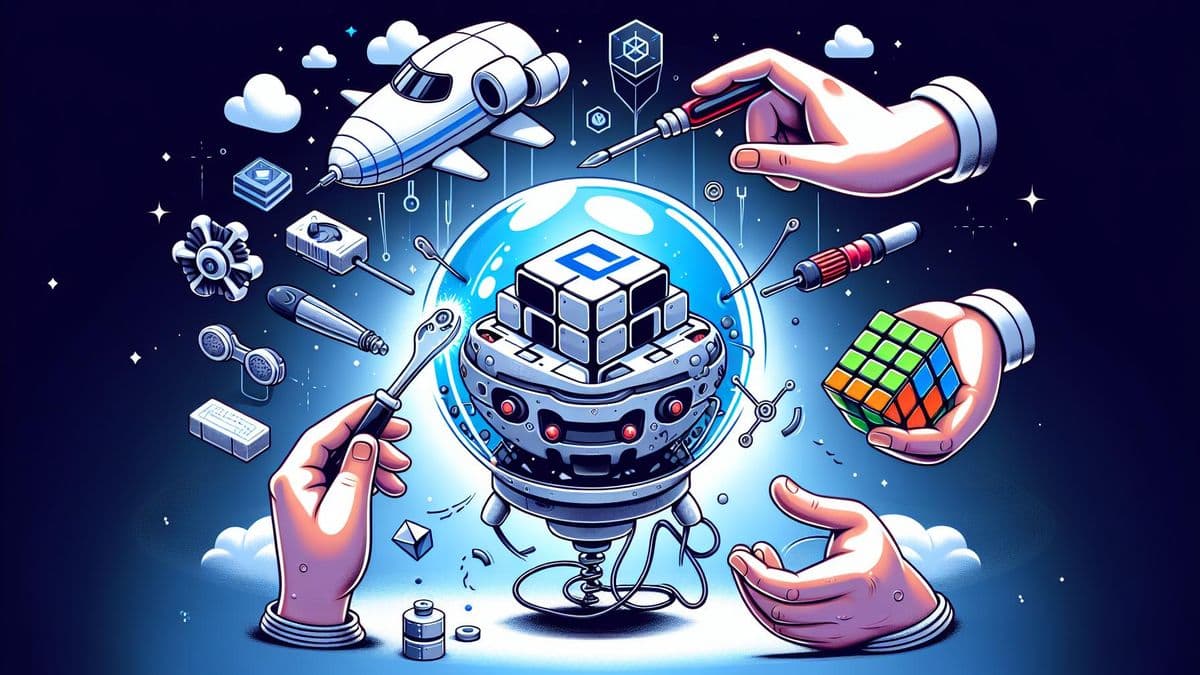
Debugging dotnet microservices deployed in kubernetes, locally.
This blog post guides full-stack software engineers through the process of locally debugging .NET microservices deployed in Kubernetes, highlighting essential tools and methodologies to streamline the process.
Debugging microservices can sometimes feel like trying to fix a spaceship with a screwdriver, especially when those microservices are crafted in .NET and deployed on Kubernetes. But fear not, dear developer! This guide will help you navigate the intricate world of local debugging without sending a constant barrage of redeployments to your beloved (or dreaded) Kubernetes cluster.
Who is this article for?
This article is tailored for full-stack software engineers who may find themselves slightly befuddled by the complexities of Kubernetes, Docker, and debugging tools. If DevOps is a hazy distant planet in your development universe, then buckle up! Weโll voyage through the essential tools and methodologies to make local debugging as seamless as solving a Rubik's cube with fingers of magic. ๐ฉโจ
Setting the Stage: Why Debug Locally?
Before we dive in, let's talk context. Debugging locally is fundamentally about:
- Speed: You make a change, you see it instantly, without the coil of redeployment.
- Resource Efficiency: Avoid unnecessary cloud costs and network overhead by keeping debugging cycles local.
- Peace of Mind: Know your code works before unleashing it into the wild Kubernetes.
Alright, let's launch into the cosmos of local debugging for .NET microservices! ๐
Tooling for Debugging .NET Microservices
Tools of the Trade: Telepresence, Gefyra, and Mirrord
To efficiently debug services locally, these amazing tools create bridges between your development environment and remote Kubernetes clusters:
-
Telepresence: Think of Telepresence as your favorite sci-fi portal. It temporarily replaces a pod in your Kubernetes cluster with code running on your local machine.
-
Gefyra: Gefyra is like a ninja - operating silently and efficiently. It allows the execution of your application components locally while they still interact with the remote cluster.
-
Mirrord: This tool mirrors traffic from the cluster to your local service, letting you debug kind of like being a superhero without a cape.
Example Code with Telepresence:
telepresence --swap-deployment <your-deployment> --run <your-dotnet-run-command>
Kubernetes in Docker: Your Local Simulators
For those grounding their interstellar ambitions locally, tools like Docker Desktop, Minikube, or kind help simulate Kubernetes clusters within your own terrestrial confines - no need for hefty infrastructure. Think of these as sandbox spaceships.
- Docker Desktop: Simple and efficient, enable Kubernetes with just a few clicks.
- Minikube: It spins up a local cluster for testing and learning, using lightweight VMs.
- kind: Orchestration using containers โ efficient like a cat in a laser show.
Setting Up a Local Kubernetes Cluster with Docker Desktop
Firstly, ensure Docker Desktop is installed. Then:
- Open Docker Desktop.
- Navigate to settings (gear icon).
- Enable Kubernetes and hit "Apply & Restart".
Now you have a local Kubernetes planet to explore! ๐
The IDE Connection: Visual Studio Code
Raise your hand if you love VS Code! ๐โโ๏ธ Perfect, because it integrates beautifully with Kubernetes. Utilize the "Bridge to Kubernetes" feature for a seamless experience.
- Bridge to Kubernetes: This allows your local code to interact directly with the Kubernetes cluster, reducing the round trips needed for code changes.
Example VS Code Configuration:
Here's how you might connect VS Code to Kubernetes:
{ "version": "1.0", "registries": { "dockerHub": { "url": "https://index.docker.io/v1/" } }, "services": { "my-dotnet-service": { "remote": true, "connect": { "service": "my-dotnet-service" } } } }
Configuring Kubernetes Components and Responding to Specific Queries
Configuring Docker for Kubernetes
To create a working local Kubernetes cluster:
- Open Docker Desktop.
- Go to the Settings and activate Kubernetes.
- Voilร ! Your local cluster emerges like a tech-savvy genie.
Managing Deployments with Skaffold
Skaffold eases the pain of the build-push-deploy cycle with automatic redeployment of code changes.
- Dev Mode: Skaffold watches your files and when they change, it triggers rebuilds โ magic code regeneration!
Running Skaffold:
skaffold dev
Configuring Nginx Ingress
Nginx Ingress is your traffic manager, directing external requests to your internal services like a maestro conducting an orchestra.
apiVersion: networking.k8s.io/v1 kind: Ingress metadata: name: example-ingress spec: rules: - host: example.com http: paths: - path: / pathType: Prefix backend: service: name: example-service port: number: 80
Hot Reloading Changes
Tools like Skaffold in dev mode provide hot-reloading, watching for file changes and redeploying faster than you can say โKubernetesโ.
Peeking into Containers: The SSH Way
With VS Code Remote - SSH, you can gain laser-precise access directly into container realms for debugging purposes.
- Ensure SSH keys and necessary permissions are properly configured.
- Use the extension to connect, and then explore and debug to your engineer's heart's content.
References to Dive Deeper
Conclusion: Debugging with Panache ๐บ
By leveraging these tools, processes, and configurations, you empower yourself to effectively manage and debug .NET microservices within Kubernetes. Remember, every problem has a solution, and understanding your toolkit is half the battle won. Your microservices can flourish like well-watered plants in this tech garden.
So, grab your debugging tools, and venture boldly into Kubernetes realms, fellow developer. May your bugs be few and your joy be endless! ๐๐
Happy coding!